Serving an HTML Page from Azure Functions
Sunday, April 10, 2016
I was playing around with building an API using Azure Functions this weekend. I wanted to see if I can host the HTML frontend for interacting with it on Azure Functions as well. Because Functions with an HTTP trigger automatically comes with an HTTP endpoint with optional built-in API key authentication (no API gateway required!), this is really easy.
Update - March 10, 2017: Check out this post on how to serve multiple files from a directory.
Create an HttpTrigger Function
If there isn't a Function App already, the first step is to go into the Azure Portal and create one. Then create an HttpTrigger C# function. This example is in .NET, but we should be able to do this with Node.js and other technologies as well.
The function is pretty simple, and it returns the HttpResponseMessage
class that we're used to in ASP.NET Web API 2. We can create an HttpResponseMessage
that has a content type of text/html
and a FileStream
of an HTML file that we'll create later. I couldn't find an elegant way of figuring out the full path to the function directory so I just hardcoded it. :) Here's the updated run.csx:
using System.Net;
using System.Net.Http.Headers;
using System.Threading.Tasks;
using System.IO;
public static HttpResponseMessage Run(HttpRequestMessage req, TraceWriter log)
{
var response = new HttpResponseMessage(HttpStatusCode.OK);
var stream = new FileStream(@"d:\home\site\wwwroot\ShoppingList\index.html", FileMode.Open);
response.Content = new StreamContent(stream);
response.Content.Headers.ContentType = new MediaTypeHeaderValue("text/html");
return response;
}
Create the HTML file
To add more files to our function app, we can use the show files and add files functionality in the Functions portal.
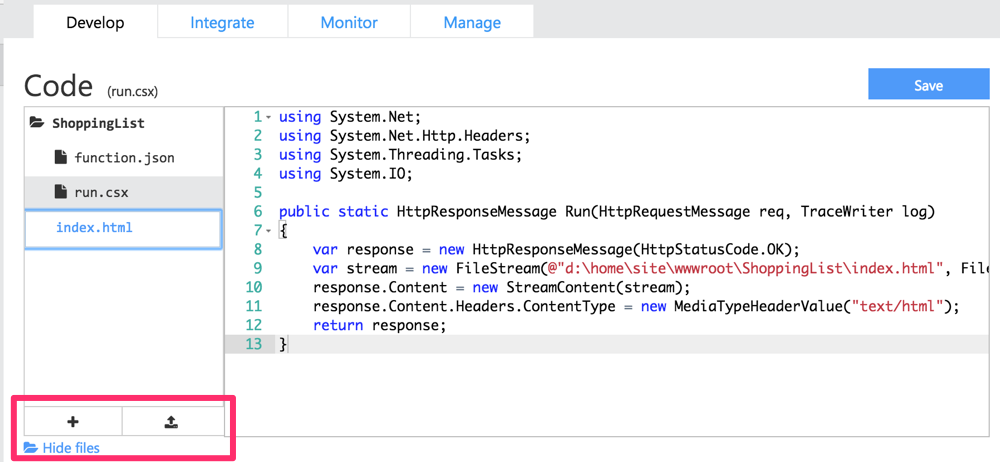
If we're using git deployment, we can simply add this file to our repo.
Here's the HTML file we'll create (index.html):
<html>
<head>
<title>hello world</title>
</head>
<body>
<h1>Hello world!</h1>
</body>
</html>
Dynamic files like Razor should work as well. We'd have to bring in the Razor templating engine to process them.
Hit the Function URL
Now visit the function's URL and we should see our HTML page:
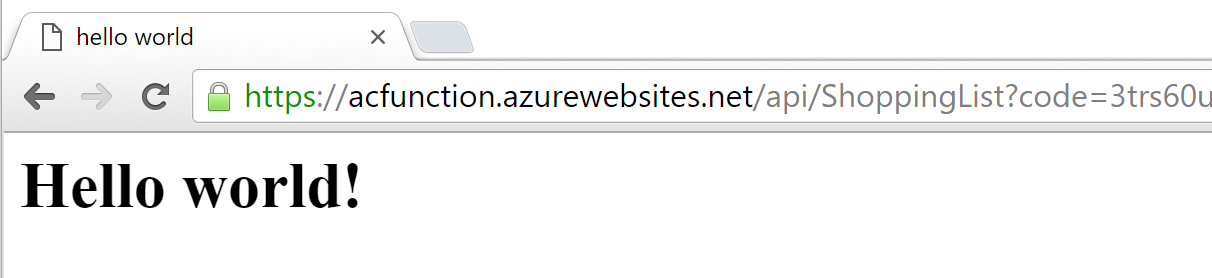
I was playing around with building an API using Azure Functions this weekend. I wanted to see if I can host the HTML frontend for interacting with it on Azure Functions as well. Because Functions with an HTTP trigger automatically comes with an HTTP endpoint with optional built-in API key authentication (no API gateway required!), this is really easy.
Update - March 10, 2017: Check out this post on how to serve multiple files from a directory.
Create an HttpTrigger Function
If there isn't a Function App already, the first step is to go into the Azure Portal and create one. Then create an HttpTrigger C# function. This example is in .NET, but we should be able to do this with Node.js and other technologies as well.
The function is pretty simple, and it returns the HttpResponseMessage
class that we're used to in ASP.NET Web API 2. We can create an HttpResponseMessage
that has a content type of text/html
and a FileStream
of an HTML file that we'll create later. I couldn't find an elegant way of figuring out the full path to the function directory so I just hardcoded it. :) Here's the updated run.csx:
using System.Net;
using System.Net.Http.Headers;
using System.Threading.Tasks;
using System.IO;
public static HttpResponseMessage Run(HttpRequestMessage req, TraceWriter log)
{
var response = new HttpResponseMessage(HttpStatusCode.OK);
var stream = new FileStream(@"d:\home\site\wwwroot\ShoppingList\index.html", FileMode.Open);
response.Content = new StreamContent(stream);
response.Content.Headers.ContentType = new MediaTypeHeaderValue("text/html");
return response;
}
Create the HTML file
To add more files to our function app, we can use the show files and add files functionality in the Functions portal.
If we're using git deployment, we can simply add this file to our repo.
Here's the HTML file we'll create (index.html):
<html>
<head>
<title>hello world</title>
</head>
<body>
<h1>Hello world!</h1>
</body>
</html>
Dynamic files like Razor should work as well. We'd have to bring in the Razor templating engine to process them.
Hit the Function URL
Now visit the function's URL and we should see our HTML page: